15 things you should be doing to make yourself a better WordPress developer
Written by September 22, 2020
onOver the years I have read a lot of WordPress code in the form of plugins and themes. Over that time I have built up a list of the 15 basic things that you should be doing to avoid the mistakes that I have seen. Here are 15 things you should be doing to make you a better developer.
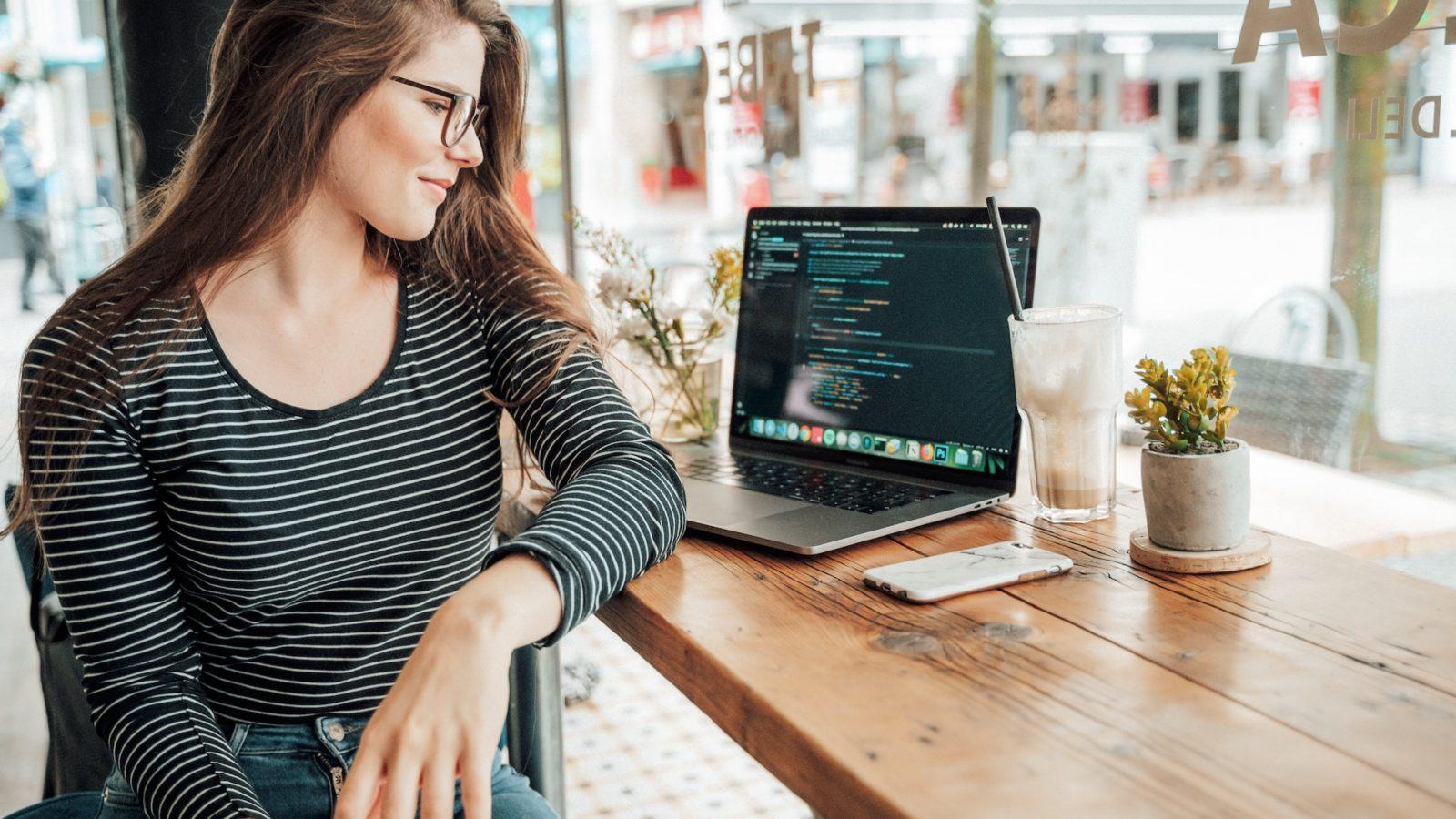
TABLE OF CONTENTS
- Comment your code
- Turn on WP_DEBUG in your development environment
- Use the Query Monitor plugin in your development environment
- Make your code neat, tidy and readable
- Follow the WordPress coding standards
- Namespace your functions and classes
- Don’t reinvent the wheel
- Make your code as extensible as possible
- Enqueue scripts and styles correctly
- Use the DRY (don’t repeat yourself) coding principles
- Use nonces when processing and submitting data
- Escape data output and sanitise data input
- Plugins should always be in their own folder
- Make all strings translatable
- Use a child theme when appropriate
1. Comment your code
I said they were in no particular order, but I must admit this is perhaps that one that bugs me most.
Please add comments to your code. Adding comments does a few things:
- It provides context to what you are trying to do
- It neatens up your code and forces you to think about what you are writing
- It tells the reader your intent – what the code should be doing
- Allows others to understand how your code works, much faster than just reading the code.
2. Turn on WP debug in your development environment
This comes from the number of times that I look at sites and they have all sorts of errors and warnings in the log files on the server. This is because the developer, when coding the plugin or theme has not had warnings and errors either being logged or better still displayed on the screen.
This is easy to do in WordPress using the following code:
// this tells WordPress to output errors and warnings.
define( 'WP_DEBUG', true );
// this logs the errors to a file called debug.log in your sites wp-content folder.
define( 'WP_DEBUG_LOG', true );
// you could set this to true to output the errors to the screen.
define( 'WP_DEBUG_DISPLAY', false );
The WP_DEBUG
constant set to true, means that WordPress will output warnings and errors. WP_DEBUG_LOG
set to true
means that these will be logged in a file called debug.log
in your sites wp-content
folder. Finally WP_DEBUG_DISPLAY
set to true
means WordPress will output these errors and warnings to the screen for you to see. I like to log them rather than print but it is up to you.
In any case, make sure that WP_DEBUG
is always set to true
when developing either locally or in a development environment.
3. Use the Query monitor plugin in your development environment
The Query Monitor WordPress plugin from John Blackbourn is an absolute must when developing for WordPress. The plugin provides a host of information to developers such as (from the website):
- Database queries
- PHP errors
- Hooks and actions
- Enqueued scripts and styles
- Theme template files
- Languages and translations
- Rewrite rules
- Block editor blocks
- HTTP API calls
- and much more…
It also highlights things such as slow queries and gives developers a great head start when looking for bottle necks in your code.
John, the developer of the plugin also writes a number of other popular a very useful plugins for WordPress. You can now support these using his new sponsors program.
4. Make your code neat, tidy and readable
There is nothing worse than opening up a PHP and the code is very hard to read, poorly spaced and not indented correctly. It makes the code hard to read and ultimately means that development takes longer.
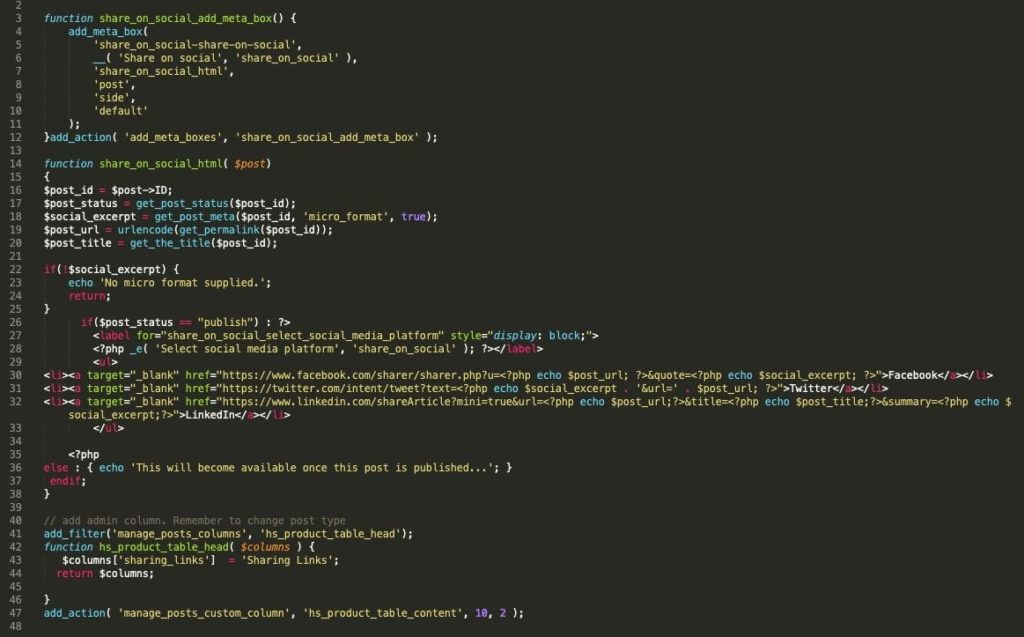
5. Follow the WordPress coding standards
The WordPress team has released a set of coding standards for developing for WordPress. These include standards for PHP, HTML, CSS and Javascript.
The standards provide guidance on the best practice for writing code in WordPress and means that everyone is working from the same set of standards to standardise WordPress development as much as possible.
The coding standards help improve the readability of code, help in the ability to catch and reduce errors and simplify the modification of code in the future.
I would highly recommend taking a look at the standards and following them as much as possible if you are developing for WordPress.
6. Namespace your functions and classes
You should always namespace you functions and classes when developing WordPress code to avoid clashes with other plugins and or themes. At the most basic level this would mean prefixing things with some letters in order to make the names of your functions and classes unique.
For example, at Highrise Digital we often prefix function names with hd_
to make sure that they are more unique.
If you are writing object orientated code then inside your classes you don’t have to namespace methods which means they look cleaner and are easier to read.
If you want to read more about namespacing, particularly at a more advanced level, Tom McFarlin has a great series called Using Namespaces and Autoloading in WordPress plugins.
7. Don’t reinvent the wheel
WordPress comes with a whole host of functions, classes and APIs for you to use. Therefore if you are looking to do something with WordPress development it is likely that WordPress already has a function, class or API that you can make use of, and you should use it, rather than writing your own.
Using WordPress functions means that should they change in the future then it is likely you won’t have to go back and re-model your code. Also WordPress functions etc. have lots of good stuff built in like caching and data sanitisation etc.
If you roll your own stuff you would need to think about all this stuff yourself!
8. Make your code as extensible as possible
Extensible code is code that can be modified or built upon without actually changing the code itself. This is good because it allows other developers to interact with and change the behaviour of your code without breaking the code itself.
Think about all the plugins you have used that do 90% of what you want or do everything but you would love to change that one thing. Extensibly developed plugins allow you to do just that.
An example of extensibility is providing developers with looks of hooks (actions and filters) to change data and run their own code at certain points during the codes execution.
If you want to learn more about writing extensible WordPress code, take a look at my article on hooks and filters or watch the video below!
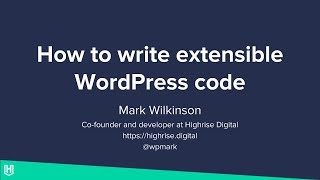
9. Enqueue scripts and styles correctly
Often many plugins and themes use the same scripts and because of this WordPress has the concept of enqueuing styles and scripts rather than just printing them in the head or footer of the page.
This means that WordPress handles outputting a script or style only once, even if multiple plugins or themes are wanting to add the same script or style. This avoids errors in your code.
To do this, use the wp_enqueue_script()
and wp_enqueue_style()
functions.
You can read more about this on the WordPress developer documentation page:
Scripts and styles should NOT be printed in the page head or foot.
10. Use the DRY (Don’t Repeat Yourself) coding principles
This is the concept of avoiding repetition in code. Repetition is inefficient and means that code is harder to maintain. This is because if you want to make changes to code in the future, without using DRY principles there could be multiple instances of the same code to change.
In WordPress, DRY principles include things such as:
- Creating functions/classes which can be re-used to carry out repetitive tasks
- Using
get_template_part()
, which you can now pass data too - Hooking code into action hooks
Learn more about WordPress DRY coding principles.
11. Use nonces when processing and submitting data
NONCE in WordPress stands for number used only once. Nonces check for a users intent rather than just the capability of whether they are allowed to do something.
They help protect against certain types of attack and therefore provide an important part of secure coding practice in WordPress.
They are a unique string of letters and numbers which have a limited lifetime. This means they will only work for a set timespan.
Find out more about using nonces here.
12. Escape data output and sanitise date input
Any data should be considered malicious and therefore it is important that we treat it as such when developing for WordPress. In practice this means not trusting user input or data we want to save to the WordPress database, or output to the browser.
When a user inputs data, for example into a form, before we save it we need to make sure that it is safe (for example no scripts injected into it) and that it is in the format we are expecting. We do this using WordPress’ sanitisation functions.
WordPress sanitisation functions
Before we output data to the browser, again we need to make sure that it is not malicious and that it is in the expected format. WordPress provides us with escaping functions which do just that, depending on the type of data you are working with.
Using these functions in your code will ensure that your code is more secure and is therefore a must for all WordPress developers.
13. Plugins should always be in their own folder
To be honest this is just something neaten up the WordPress plugins folder, but also has benefits for if you want to change your plugin file structure in the future.
Always place a plugin in its own folder in the WordPress plugins folder rather than directly in the root. This is for two reasons:
- It makes the folder neat and tidy and easy to scan the list of folders (plugins) present
- If a developer wants to add more files to a plugin in the future they would need to place these inside a folder. Doing so would then deactivate a plugin on a site, or cause it to load twice because WordPress stores the path of active plugins, referenced from the plugins folder root.
14. Make all strings translatable
When we output strings of text in our code these should always be translatable into different languages so that users running WordPress in another language can use a translation file to read the strings in their own language.
This is relatively easy to do as WordPress provides a suite of functions we can use as developers to allow our strings to be translatable.
To learn more how to do this I would highly recommend watching John Blackbourn’s presentation at WordPress Bristol about Internationalisation for WordPress Developers.
15. Use a child theme when appropriate
If you are using a WordPress theme which receives updates from time to time you should certainly consider running a child theme of that theme on your site rather than the theme itself.
The reason for this is so that you can make modifications to the theme without having them overwritten with future updates.
Watch me explain this in an AskHighrise episode on Commercial themes and using a child theme.
Have I missed any obvious ones? Let me know on Twitter if you think I need to add something to this list!