Escaping data in WordPress
Written by October 5, 2020
onEscaping data when building WordPress plugins and themes is super important for security. This post outlines the main functions or methods which developers can use in order to make sure they code is escaped properly.
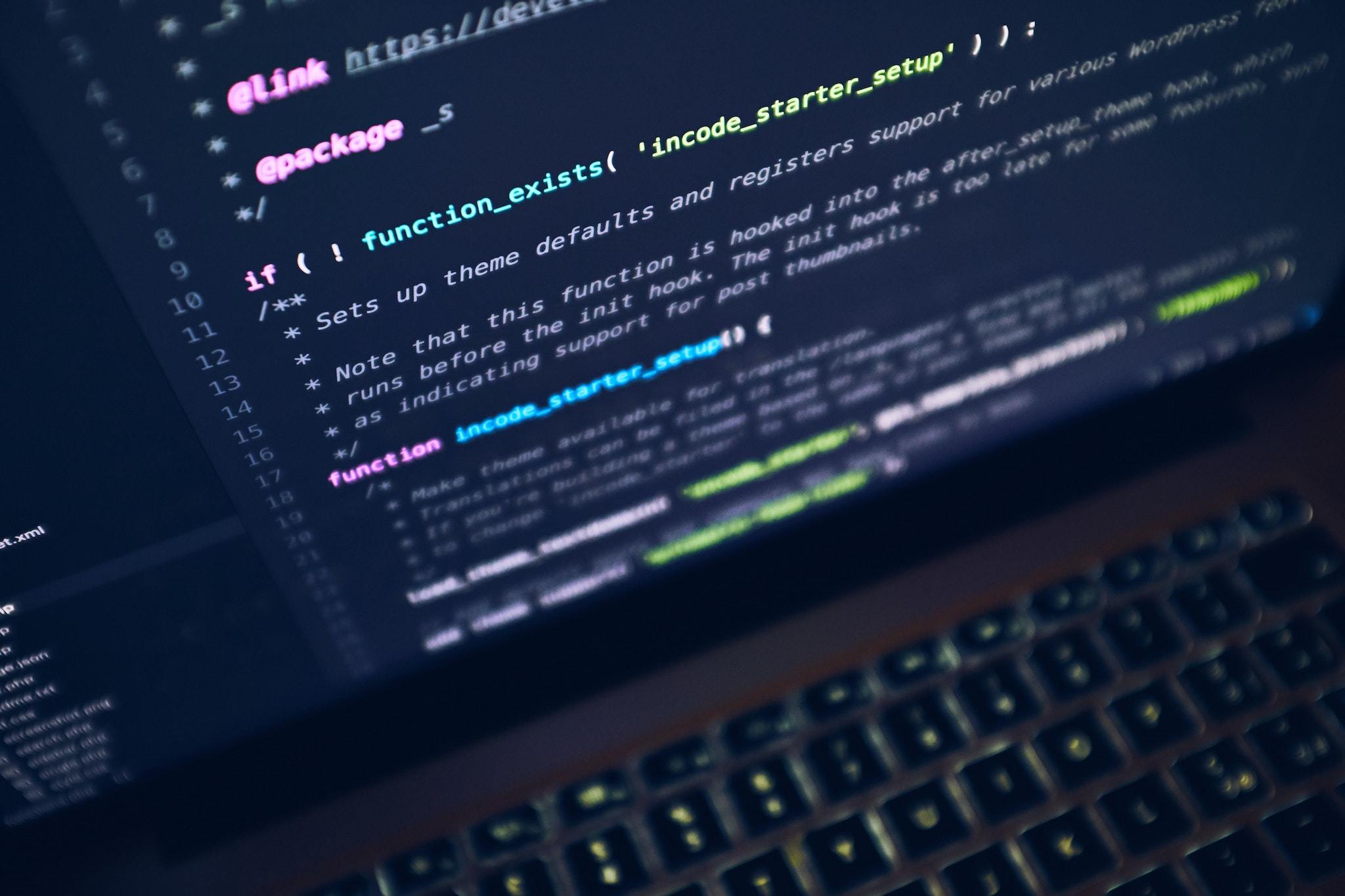
Escaping data is the process of securing output by stripping any unwanted data such as script tags, incorrectly formed HTML and other unwanted data. It therefore prevents of this data being seen or executed as code.
Escaping data is a crucial part of secure coding in WordPress and should be considered for all WordPress development.
Thankfully, WordPress core provides developers with a suite of functions or methods which can be used to escape data for us. We just need to learn which is the correct function to use, depending of what data we are using and where we are outputting it.
In this post let’s take a look at the functions available and when to use each one.
esc_html()
We are often working with variables that we want to output inside HTML elements. A good example of this is perhaps when you retrieve the title of something from the database and then you want to output this inside a heading tag.
At this point we need to make sure that the output does not contain for example a closing heading tag. If this was the case then it would close our heading tag and break the HTML markup of the page.
Therefore we need to escape any HTML that may be in the title to make sure that the HTML markup and layout doesn’t break. This is where the esc_html()
function is used. It will make sure that the output has all HTML escaped and therefore not treated as HTML.
Take a look at the simple example below.
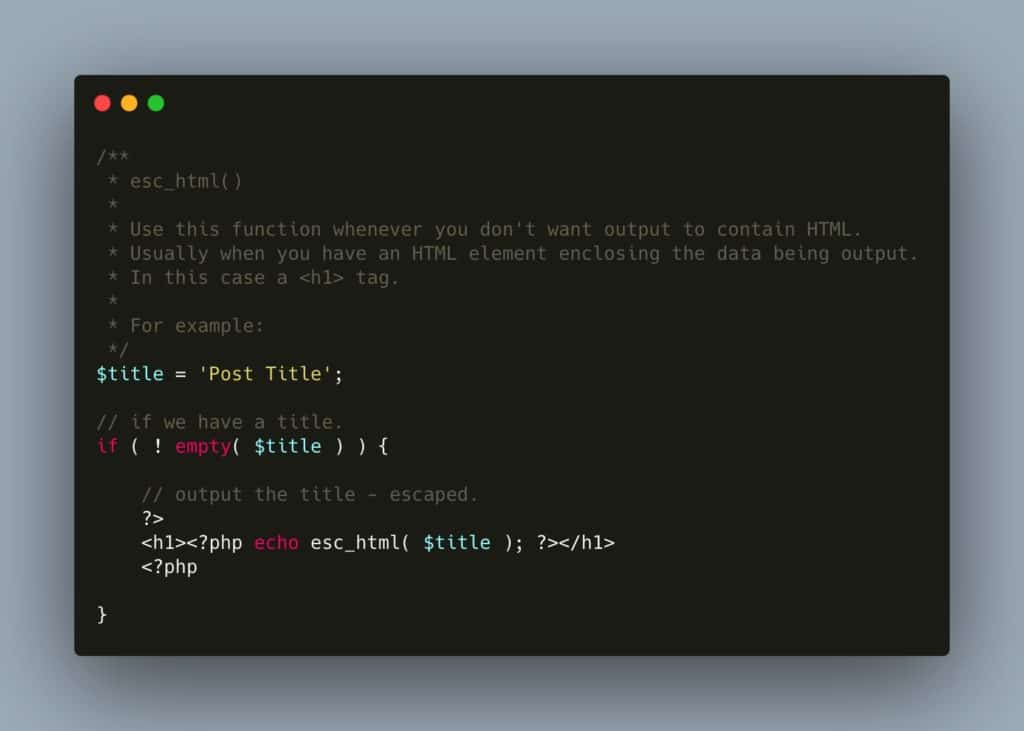
wp_kses()
Sometimes however there are times when you might want output inside an HTML element that will contain specific allowed HTML. A great example of this is when you have content added to the WordPress post or block editor. This is likely to contain images, paragraphs, bold text and of course links.
In this case we want to allow specific HTML and disallow HTML that we know is not safe and has the potential to cause problems, such as script tags etc.
The wp_kses()
function allows us to pass in the string we want escaping correctly (in this case the post content), an array of allowed HTML elements (or the name of a predefined set) and an array of allowed protocols.
The function also has some wrapper functions which make it easier to use. Perhaps the most used and best know is the wp_kses_post()
function which use the allowed HTML and protocols for post content without the need to specify them.
Here is a simple example of how this would be used.
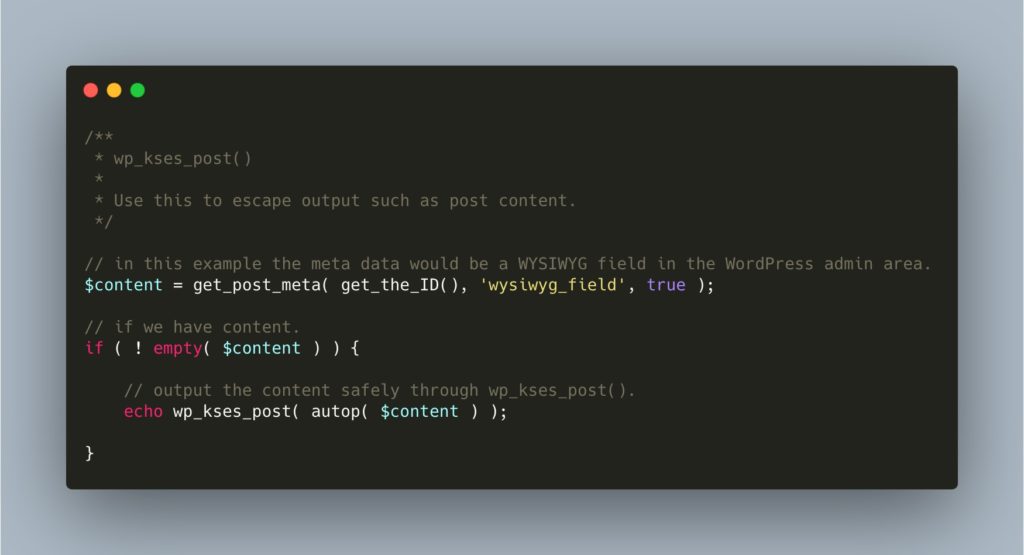
Note the use of the WordPress function wpautop()
which adds paragraph tags to a WYSIWYG fields entry.
esc_url()
We may want to output a variable to be a URL, perhaps in the src
attribute of an image or the href
part of a link. Here we need to make sure that the URL is escaped and unsafe data removed before the output.
To do this we use the esc_url()
function which makes sure the resulting output is safe to use in these instances. For example:
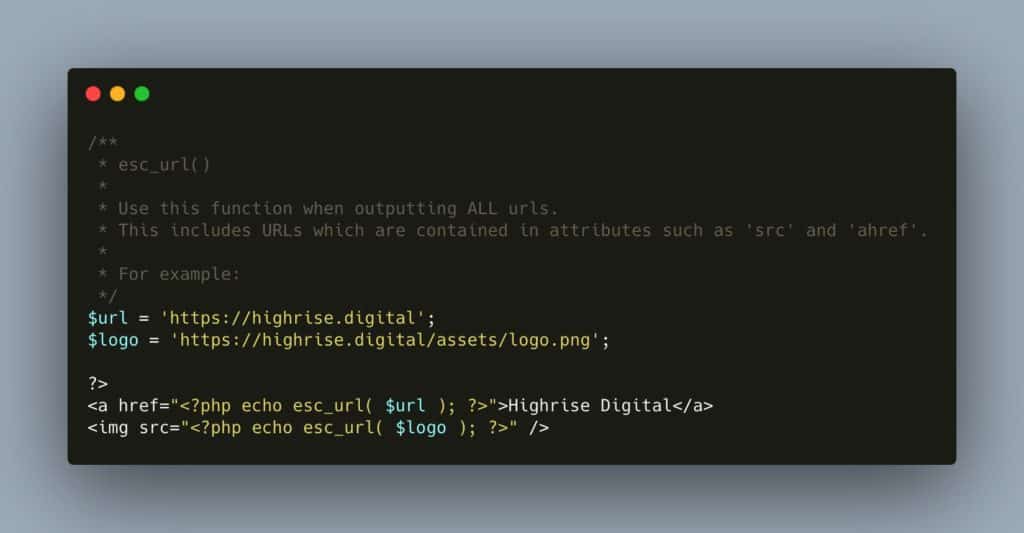
esc_js()
Often we want to add some dynamic javascript to a variable that may change depending on different things. When we want to output this javascript, inline to the page we need to make sure that is escaped to avoid any unwanted code running.
To do this we use the WordPress function named esc_js()
. Below is a simple example:
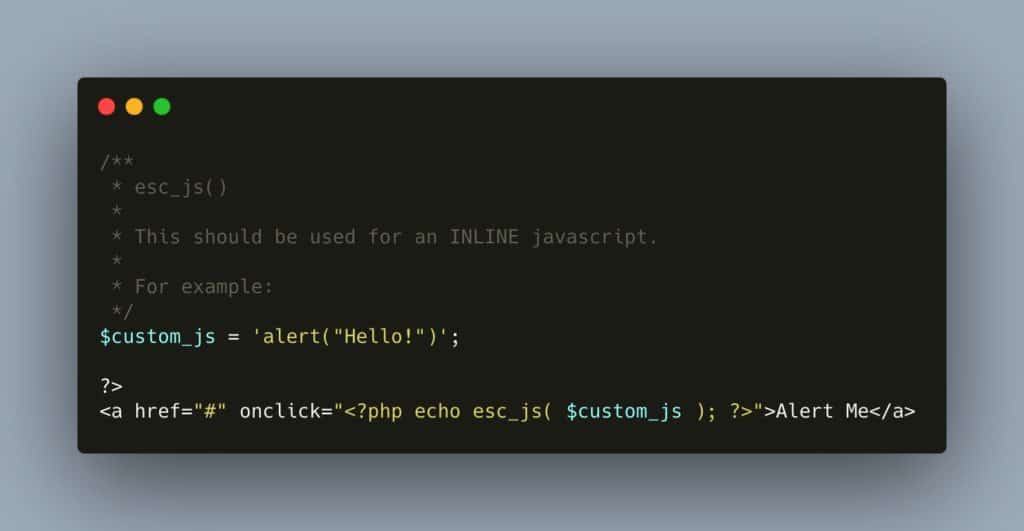
esc_attr()
Anytime that you want to output PHP into an HTML elements attributes (except if it is a URL – see above), then this must be escaped too. The correct function for doing this is the esc_attr()
function. This makes sure the output is safe for inside an attribute.
An example of using this function is below when we are dealing with classes.
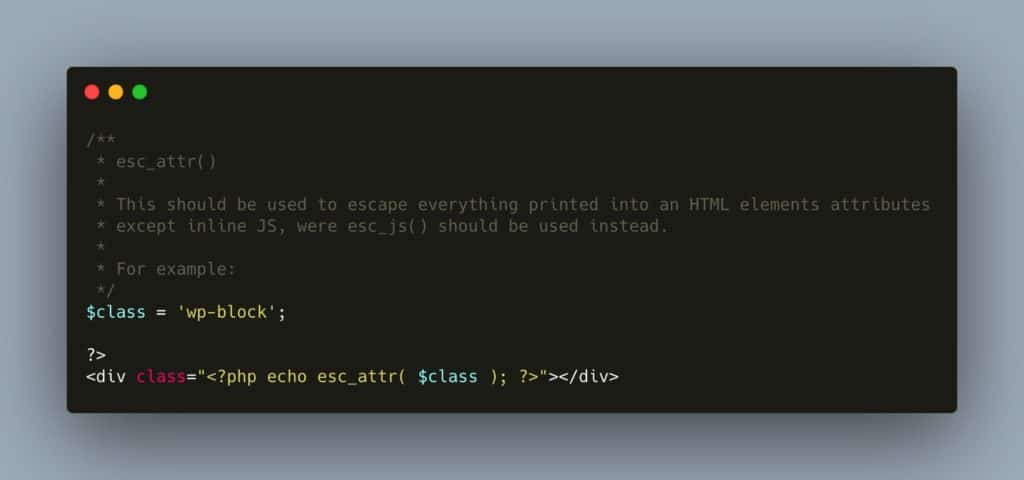
esc_textarea()
This one has a specific use case when you have data being entered into a textarea
and you want to display the saved information inside the textarea
HTML element.
Here is a simple example.
esc_html_e() and esc_attr_e()
WordPress also provides some wrapper functions for us to escape strings which also need localisation. These escaping function also include the localisations functions in them so we don’t need to nest function. For example:
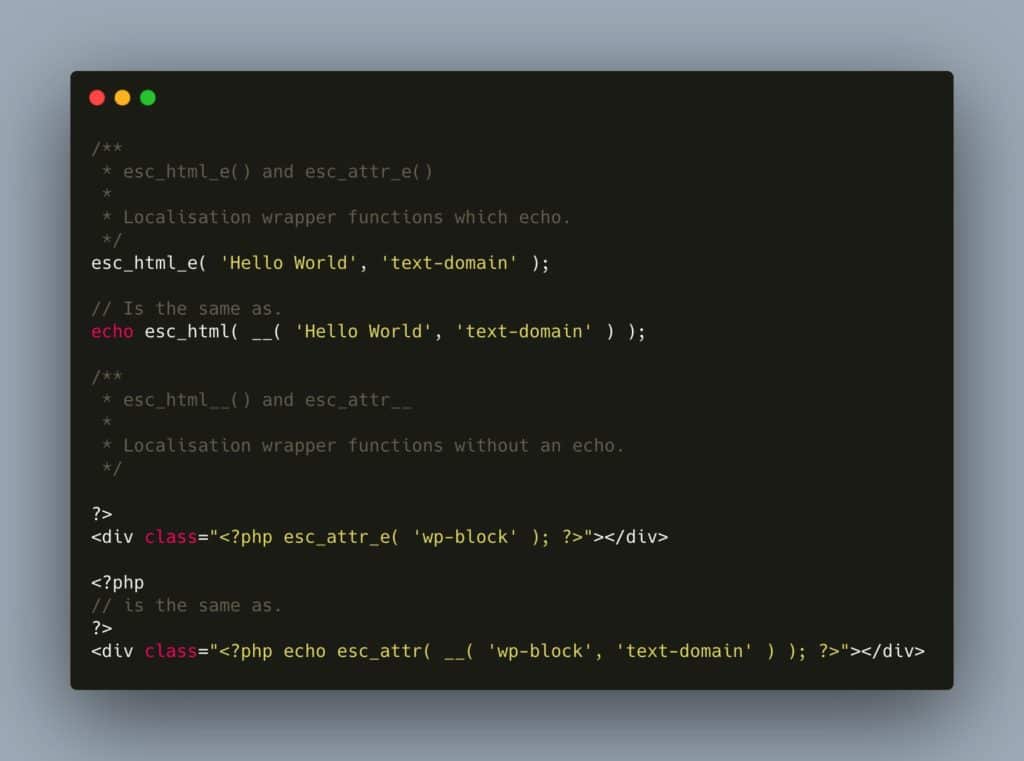
It is important to note here that these functions cannot be used with PHP variables, only with text strings that can be translated.
There you have it! A whistle stop tour of the escaping functions available to developers in WordPress, including some simple code examples. I hope you find that useful.