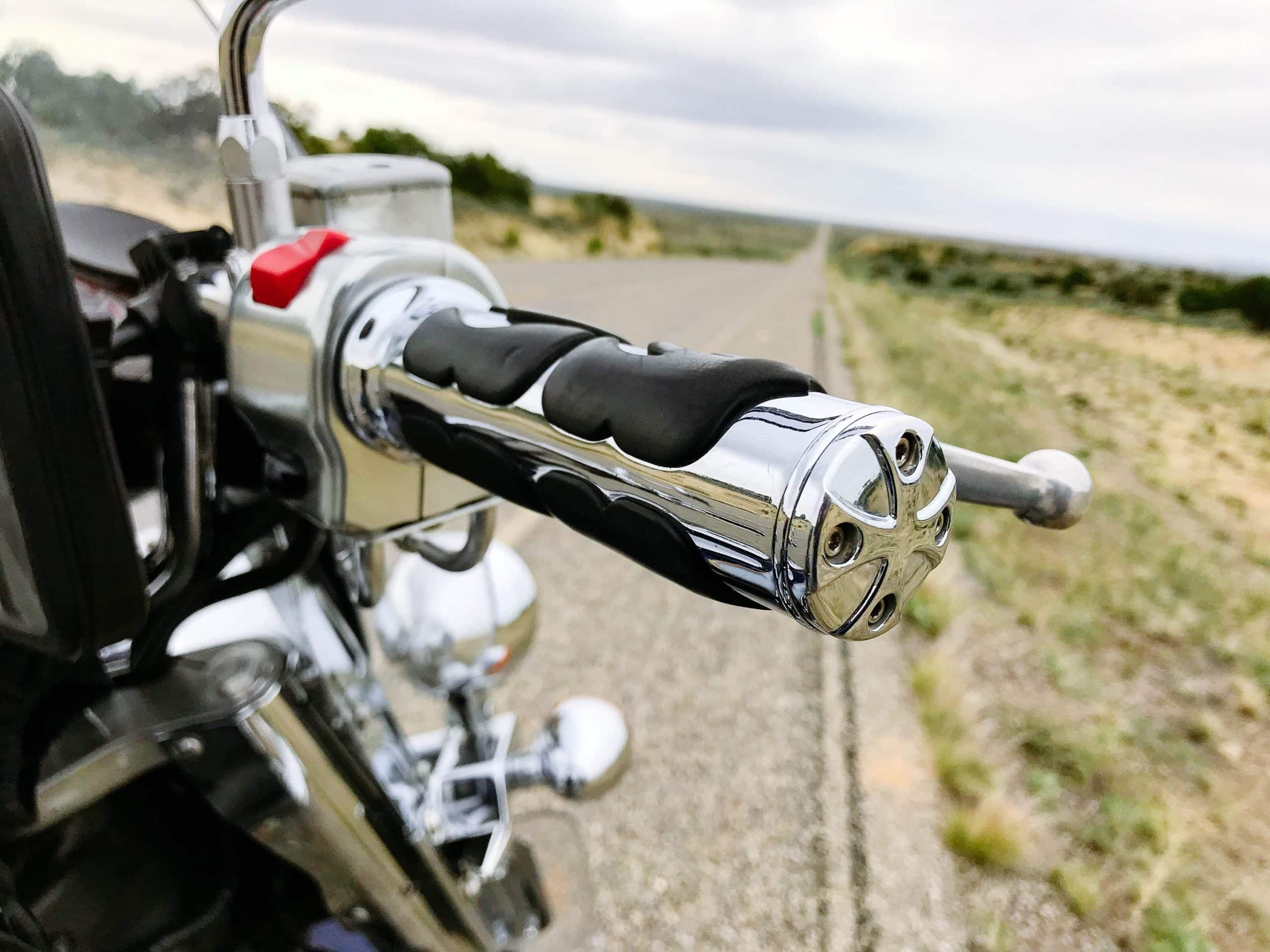
Is your front-end performance suffering from ‘jank’?
If you have functions attached to event listeners in JS, such as ‘scroll’ or ‘resize’, these will fire continuously as the event occurs.
This can have a negative effect on front-end performance, and you might start to notice some ‘jank’ on your scrolling and/or your animations.
Jank refers to sluggishness in a user interface, usually caused by executing long tasks on the main thread, blocking rendering, or expending too much processor power on background processes.
https://developer.mozilla.org/en-US/docs/Glossary/Jank
It’s unlikely that you actually need your function to run as often as your browser can manage. Therefore, what you need is something that can limit, or ‘throttle, the frequency at which your function runs.
Introducing ‘throttling’.
What is throttling in JS?
Throttling enforces a maximum number of times a function can be called over time. As in “execute this function at most once every 100 milliseconds.”
CSS Tricks
‘Throttle’ is a close cousin of ‘debounce’, and the difference between these is explained in this CSS Tricks article.
Throttling the scroll function
In my case, I wanted to limit the execution of a function on scroll and therefore ‘throttling’ was more appropriate than a ‘debounce’.
After some research, I found this fiddle that demonstrates a simple throttle function on the ‘resize’ event. I repurposed this for my needs as follows.
// our simple throttle function
function throttle (callback, limit) {
var wait = false; // Initially, we're not waiting
return function () { // We return a throttled function
if (!wait) { // If we're not waiting
callback.call(); // Execute users function
wait = true; // Prevent future invocations
setTimeout(function () { // After a period of time
wait = false; // And allow future invocations
}, limit);
}
}
}
// the function that you want to be throttled
function doStuff(){
// do some stuff
}
// On scroll, allow function to run at most 1 time per 100ms
window.addEventListener("scroll", throttle(doStuff, 100));
You can replace ‘scroll’ with ‘resize’ or any other event listener.
This is working for me, but do you know a better way? Tell us on Twitter!
(Photo by Donald Giannatti on Unsplash)